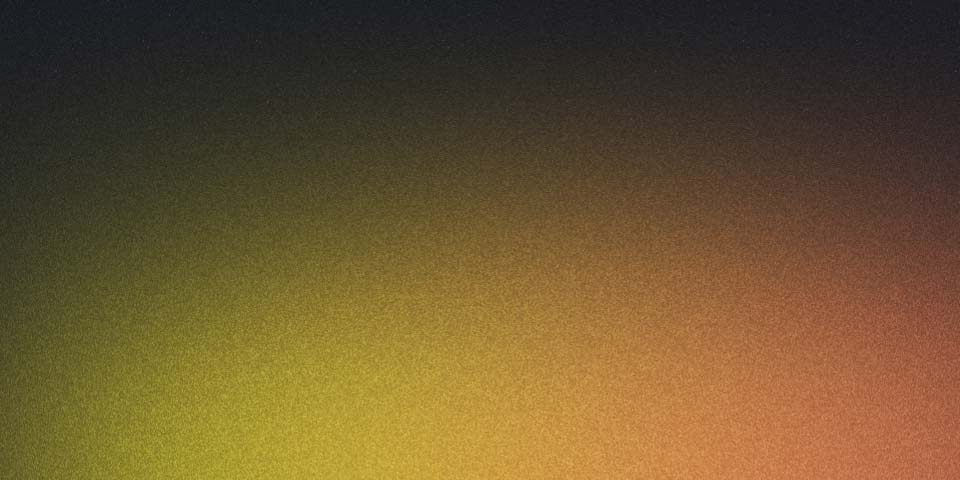
Building Scalable Microservices with Node.js
Introduction
In this post, we’ll explore how to build scalable microservices using Node.js and modern cloud technologies.
Architecture Overview
Here’s a basic example of a microservice setup:
// User Service
import express from 'express';
import mongoose from 'mongoose';
const app = express();
// Database connection
mongoose.connect('mongodb://localhost/users');
// User model
const User = mongoose.model('User', {
name: String,
email: String,
role: String
});
// API endpoints
app.get('/users', async (req, res) => {
const users = await User.find();
res.json(users);
});
app.listen(3000, () => {
console.log('User service running on port 3000');
});
Docker Configuration
Here’s how we containerize our service:
FROM node:18-alpine
WORKDIR /app
COPY package*.json ./
RUN npm install
COPY . .
EXPOSE 3000
CMD ["npm", "start"]
Load Testing Results
Load testing results showing response times under different loads
Video Tutorial
Key Learnings
- Always implement proper error handling
- Use circuit breakers for service communication
- Implement proper monitoring and logging
- Design with scalability in mind